[スポンサーリンク]
Python3でTwitterAPI使用する準備
Python3でTwitterAPI使用する準備として、下記を行いました
①APIキーとトークンの取得
②ライブラリのインストール
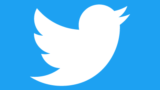
[スポンサーリンク]
ツイートを検索 Standard search API
TwitterAPIでツイートを検索するには、Standard search APIを使用します
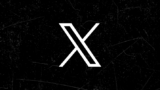
パラメータ
Standard search APIのパラメータは以下です
各項目について、メモを残しておきます
記載内容一切保証出来ません
項目名 | 要否 | 個人的メモ |
q | required | 検索対象の検索条件を指定。UTF-8で500文字上限 |
geocode | optional | 検索対象のツイート住所、地域をジオコードで指定 |
lang | optional | 検索対象の言語を指定。日本の場合デフォルトで日本語 |
locale | optional | 検索対象の地域を指定。日本の場合デフォルトで日本 |
result_type | optional | 検索タイプを指定。最新、人気、混合から選択 |
count | optional | 検索ツイート数を指定。1ページ上限100ツイート |
until | optional | 検索対象の日時上限をYYYY-MM-DDで指定 |
since_id | optional | 検索対象のツイートIDの最小IDを指定 |
max_id | optional | 検索対象のツイートIDの最大IDを指定 |
include_entities | optional | 検索対象のエンティティを指定※1 |
※1 Twitter entities
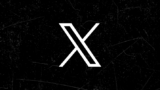
Standard search API サンプルコード
サンプルとして、TwitterJapanアカウントのツイートを2件取得します
q項目の「from:TwitterJP」で、TwitterJapanアカウントのツイートを検索条件にします
count項目の「2」で、2件のツイートを取得数と指定します
q項目については、検索条件を指定出来ます
TwitterのWEBやアプリでも、検索でも使えるの覚えていると便利です
下記サイトにて詳しく解説されています
・Standard search APIのURLを設定
1 |
StandardSearchApiUrl = 'https://api.twitter.com/1.1/search/tweets.json' |
・OAuth認証でTwitterとセッション接続
1 |
StandardSearchApiSession = OAuth1Session(ConsumerAPIKey, ConsumerAPIsecretkey, AccsessToken, AceestokenSecret) |
・Sessionクラスのgetを使って、Standard search APIのレスポンスを取得
1 |
StandardSearchResponse = StandardSearchApiSession.get(StandardSearchApiUrl, params = StandardSearchParameters) |
・SessionのHTTPのレスポンスコードが200(正常)かを判定
1 |
if StandardSearchResponse.status_code == 200 : |
・json形式として読込
1 |
json.loads(StandardSearchResponse.text) |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
# coding: utf_8 # ライブラリインポート import json from requests_oauthlib import OAuth1Session # APIキー&トークン設定 ConsumerAPIKey = 【ConsumerKey】 ConsumerAPIsecretkey = 【ConsumerSecretKey】 AccsessToken = 【AccessToken】 AceestokenSecret = 【AccessTokenSecret】 # 検索パラメータ設定 StandardSearchParameters = { 'q' : 'from:TwitterJP' ,'geocode' : '' ,'lang' : '' ,'locale' : '' ,'result_type' : '' ,'count' : '2' ,'until' : '' ,'since_id' : '' ,'max_id' : '' ,'include_entities' : '' } StandardSearchApiSession = OAuth1Session(ConsumerAPIKey, ConsumerAPIsecretkey, AccsessToken, AceestokenSecret) StandardSearchApiUrl = 'https://api.twitter.com/1.1/search/tweets.json' # 検索実行 StandardSearchResponse = StandardSearchApiSession.get(StandardSearchApiUrl, params = StandardSearchParameters) # 検索結果取得 if StandardSearchResponse.status_code == 200: StandardSearchResult = json.loads(StandardSearchResponse.text) print (StandardSearchResult) |
Standard search API レスポンス
Json形式でのレスポンスが返ってきます
1 |
{'statuses': [{'created_at': 'Wed Apr 22 07:08:48 +0000 2020', 'id': 1252856865479745538, 'id_str': '1252856865479745538', 'text': 'RT @Kantei_Saigai: 【注意喚起】間もなくゴールデンウィークを迎えます。#新型コロナウイルス の感染拡大を防ぐため、人との接触を避けてください。\n・帰省、旅行を控える\n・近場の外出でも密集・密接を避ける\n・買物の際は少人数・最小限の回数に\n医療現場や多くの命を守…', 'truncated': False, 'metadata': {'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': {'id': 7080152, 'id_str': '7080152', 'name': 'Twitter Japan', 'screen_name': 'TwitterJP', 'location': '東京都中央区', 'description': '日本語版Twitter公式アカウントです。サービスに関してのご質問、お問い合わせは https://t.co/mfQkUQLUhe および https://t.co/NZ3xoejW5Zをご利用ください。', 'url': 'https://t.co/veCH4MJbYL', 'entities': {'url': {'urls': [{'url': 'https://t.co/veCH4MJbYL', 'expanded_url': 'https://blog.twitter.com/ja_jp.html', 'display_url': 'blog.twitter.com/ja_jp.html', 'indices': [0, 23]}]}, 'description': {'urls': [{'url': 'https://t.co/mfQkUQLUhe', 'expanded_url': 'https://support.twitter.com/', 'display_url': 'support.twitter.com', 'indices': [42, 65]}, {'url': 'https://t.co/NZ3xoejW5Z', 'expanded_url': 'https://support.twitter.com/articles/486421', 'display_url': 'support.twitter.com/articles/486421', 'indices': [70, 93]}]}}, 'protected': False, 'followers_count': 2288941, 'friends_count': 140, 'listed_count': 15815, 'created_at': 'Tue Jun 26 01:54:35 +0000 2007', 'favourites_count': 988, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 13472, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': True, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/7080152/1493927138', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': 'FFFFFF', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': True, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'regular'}, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'retweeted_status': {'created_at': 'Wed Apr 22 07:07:13 +0000 2020', 'id': 1252856469701066753, 'id_str': '1252856469701066753', 'text': '【注意喚起】間もなくゴールデンウィークを迎えます。#新型コロナウイルス の感染拡大を防ぐため、人との接触を避けてください。\n・帰省、旅行を控える\n・近場の外出でも密集・密接を避ける\n・買物の際は少人数・最小限の回数に\n医療現場や多… https://t.co/1JH7c0fQGb', 'truncated': True, 'metadata': {'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': {'id': 265205959, 'id_str': '265205959', 'name': '首相官邸(災害・危機管理情報)', 'screen_name': 'Kantei_Saigai', 'location': '', 'description': '本アカウントは首相官邸の公式アカウントです。\n首相官邸から災害・危機管理関連の政府活動情報をお届けいたします。', 'url': 'https://t.co/YlmtZlwbSB', 'entities': {'url': {'urls': [{'url': 'https://t.co/YlmtZlwbSB', 'expanded_url': 'https://www.kantei.go.jp/', 'display_url': 'kantei.go.jp', 'indices': [0, 23]}]}, 'description': {'urls': []}}, 'protected': False, 'followers_count': 2836710, 'friends_count': 20, 'listed_count': 45036, 'created_at': 'Sun Mar 13 05:22:07 +0000 2011', 'favourites_count': 0, 'utc_offset': None, 'time_zone': None, 'geo_enabled': False, 'verified': True, 'statuses_count': 5741, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875550692454289408/Zfr37OhA_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875550692454289408/Zfr37OhA_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/265205959/1355386027', 'profile_link_color': '0084B4', 'profile_sidebar_border_color': 'C0DEED', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none'}, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': False, 'retweet_count': 5169, 'favorite_count': 5210, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja'}, 'is_quote_status': False, 'retweet_count': 5169, 'favorite_count': 0, 'favorited': False, 'retweeted': False, 'lang': 'ja'}, {'created_at': 'Wed Apr 22 07:03:31 +0000 2020', 'id': 1252855534895521793, 'id_str': '1252855534895521793', 'text': 'RT @MofaJp_Sports: フィギュアスケート羽生結弦選手からのメッセージです????\nA video message from Hanyu Yuzuru, a Japanese figure skater. (Japanese only) \n#うちで過ごそう #St…', 'truncated': False, 'metadata': {'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': {'id': 7080152, 'id_str': '7080152', 'name': 'Twitter Japan', 'screen_name': 'TwitterJP', 'location': '東京都中央区', 'description': '日本語版Twitter公式アカウントです。サービスに関してのご質問、お 問い合わせは https://t.co/mfQkUQLUhe および https://t.co/NZ3xoejW5Zをご利用ください。', 'url': 'https://t.co/veCH4MJbYL', 'entities': {'url': {'urls': [{'url': 'https://t.co/veCH4MJbYL', 'expanded_url': 'https://blog.twitter.com/ja_jp.html', 'display_url': 'blog.twitter.com/ja_jp.html', 'indices': [0, 23]}]}, 'description': {'urls': [{'url': 'https://t.co/mfQkUQLUhe', 'expanded_url': 'https://support.twitter.com/', 'display_url': 'support.twitter.com', 'indices': [42, 65]}, {'url': 'https://t.co/NZ3xoejW5Z', 'expanded_url': 'https://support.twitter.com/articles/486421', 'display_url': 'support.twitter.com/articles/486421', 'indices': [70, 93]}]}}, 'protected': False, 'followers_count': 2288941, 'friends_count': 140, 'listed_count': 15815, 'created_at': 'Tue Jun 26 01:54:35 +0000 2007', 'favourites_count': 988, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 13472, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': True, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/7080152/1493927138', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': 'FFFFFF', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': True, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'regular'}, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'retweeted_status': {'created_at': 'Tue Apr 21 10:54:13 +0000 2020', 'id': 1252551207186386944, 'id_str': '1252551207186386944', 'text': 'フィギュアスケート羽生結弦選手からのメッセージです????\nA video message from Hanyu Yuzuru, a Japanese figure skater. (Japanese only)… https://t.co/dLkQH48VEo', 'truncated': True, 'metadata': {'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': {'id': 1153493906593501184, 'id_str': '1153493906593501184', 'name': '外務省MofaJapan×SPORTS', 'screen_name': 'MofaJp_Sports', 'location': 'Tokyo,Japan', 'description': 'SPORTS account of Ministry of Foreign Affairs of Japan. \n外務省のスポーツアカウント。スポーツ交流など外務省のスポーツを通じた様々な国際的取組みについて発信します。スポーツを通して外交を身近に!', 'url': 'https://t.co/E8nLgiaEbB', 'entities': {'url': {'urls': [{'url': 'https://t.co/E8nLgiaEbB', 'expanded_url': 'https://www.mofa.go.jp/mofaj/p_pd/ep/page24_000800.html', 'display_url': 'mofa.go.jp/mofaj/p_pd/ep/…', 'indices': [0, 23]}]}, 'description': {'urls': []}}, 'protected': False, 'followers_count': 2106, 'friends_count': 284, 'listed_count': 57, 'created_at': 'Tue Jul 23 02:35:52 +0000 2019', 'favourites_count': 564, 'utc_offset': None, 'time_zone': None, 'geo_enabled': False, 'verified': True, 'statuses_count': 728, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': '000000', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/1217617971968741376/P0_I6cKy_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/1217617971968741376/P0_I6cKy_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/1153493906593501184/1578453418', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': '000000', 'profile_sidebar_fill_color': '000000', 'profile_text_color': '000000', 'profile_use_background_image': False, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none'}, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': True, 'quoted_status_id': 1251022418094649345, 'quoted_status_id_str': '1251022418094649345', 'quoted_status': {'created_at': 'Fri Apr 17 05:39:22 +0000 2020', 'id': 1251022418094649345, 'id_str': '1251022418094649345', 'text': 'フィギュアスケートの羽生結弦選手からのメッセージです??\n#いまスポーツに できること #SportsAssistYou #Staystrong #Stayhome https://t.co/ASplkSQW7V', 'truncated': False, 'metadata': {'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': {'id': 339200824, 'id_str': '339200824', 'name': '日本オリンピック委員会(JOC)', 'screen_name': 'Japan_Olympic', 'location': 'Tokyo, JAPAN', 'description': '日本オリンピック委員会(JOC)の公式ツイッターです。 Facebook: https://t.co/xgdGriW0ad Instagram: https://t.co/vb3VwM0hGF', 'url': 'https://t.co/LhygocfCSr', 'entities': {'url': {'urls': [{'url': 'https://t.co/LhygocfCSr', 'expanded_url': 'https://www.joc.or.jp/', 'display_url': 'joc.or.jp', 'indices': [0, 23]}]}, 'description': {'urls': [{'url': 'https://t.co/xgdGriW0ad', 'expanded_url': 'https://www.facebook.com/JapanOlympicTeam/', 'display_url': 'facebook.com/JapanOlympicTe…', 'indices': [38, 61]}, {'url': 'https://t.co/vb3VwM0hGF', 'expanded_url': 'https://www.instagram.com/team_nippon/', 'display_url': 'instagram.com/team_nippon/', 'indices': [73, 96]}]}}, 'protected': False, 'followers_count': 413875, 'friends_count': 534, 'listed_count': 2227, 'created_at': 'Wed Jul 20 18:52:37 +0000 2011', 'favourites_count': 1485, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 14321, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': '9AE4E8', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme16/bg.gif', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme16/bg.gif', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875523921675665408/TDOMhMgV_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875523921675665408/TDOMhMgV_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/339200824/1580371416', 'profile_link_color': '0084B4', 'profile_sidebar_border_color': 'BDDCAD', 'profile_sidebar_fill_color': 'DDFFCC', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none'}, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': False, 'retweet_count': 14199, 'favorite_count': 30221, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja'}, 'retweet_count': 1069, 'favorite_count': 2607, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja'}, 'is_quote_status': True, 'quoted_status_id': 1251022418094649345, 'quoted_status_id_str': '1251022418094649345', 'retweet_count': 1069, 'favorite_count': 0, 'favorited': False, 'retweeted': False, 'lang': 'ja'}], 'search_metadata': {'completed_in': 0.032, 'max_id': 0, 'max_id_str': '0', 'next_results': '?max_id=1252855534895521792&q=from%3ATwitterJP&geocode=&lang=&count=2&result_type=', 'query': 'from%3ATwitterJP', 'count': 2, 'since_id': 0, 'since_id_str': '0'}} |
dict(辞書)型とlist(リスト)型で構成されています
これを、整形しています
かなりの情報量があります(汗)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 |
{ 'statuses': [ { 'created_at': 'Wed Apr 22 07:08:48 +0000 2020', 'id': 1252856865479745538, 'id_str': '1252856865479745538', 'text': 'RT @Kantei_Saigai: 【注意喚起】間もなくゴールデンウィークを迎えます。#新型コロナウイルス の感染拡大を防ぐため、人との接触を避けてください。\n・帰省、旅行を控える\n・近場の外出でも密集・密接を避ける\n・買物の際は少人数・最小限の回数に\n医療現場や多くの命を守…', 'truncated': False, 'metadata': { 'iso_language_code': 'ja', 'result_type': 'recent'}, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': { 'id': 7080152, 'id_str': '7080152', 'name': 'Twitter Japan', 'screen_name': 'TwitterJP', 'location': '東京都中央区', 'description': '日本語版Twitter公式アカウントです。サービスに関してのご質問、お問い合わせは https://t.co/mfQkUQLUhe および https://t.co/NZ3xoejW5Zをご利用ください。', 'url': 'https://t.co/veCH4MJbYL', 'entities': { 'url': { 'urls': [ { 'url': 'https://t.co/veCH4MJbYL', 'expanded_url': 'https://blog.twitter.com/ja_jp.html', 'display_url': 'blog.twitter.com/ja_jp.html', 'indices': [0, 23] } ] }, 'description': { 'urls': [ { 'url': 'https://t.co/mfQkUQLUhe', 'expanded_url': 'https://support.twitter.com/', 'display_url': 'support.twitter.com', 'indices': [42, 65] }, { 'url': 'https://t.co/NZ3xoejW5Z', 'expanded_url': 'https://support.twitter.com/articles/486421', 'display_url': 'support.twitter.com/articles/486421', 'indices': [70, 93] } ] } }, 'protected': False, 'followers_count': 2288941, 'friends_count': 140, 'listed_count': 15815, 'created_at': 'Tue Jun 26 01:54:35 +0000 2007', 'favourites_count': 988, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 13472, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': True, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/7080152/1493927138', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': 'FFFFFF', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': True, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'regular' }, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'retweeted_status': { 'created_at': 'Wed Apr 22 07:07:13 +0000 2020', 'id': 1252856469701066753, 'id_str': '1252856469701066753', 'text': '【注意喚起】間もなくゴールデンウィークを迎えます。#新型コロナウイルス の感染拡大を防ぐため、人との接触を避けてください。\n・帰省、旅行を控える\n・近場の外出でも密集・密接を避ける\n・買物の際は少人数・最小限の回数に\n医療現場や多… https://t.co/1JH7c0fQGb', 'truncated': True, 'metadata': { 'iso_language_code': 'ja', 'result_type': 'recent' }, 'source': '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': { 'id': 265205959, 'id_str': '265205959', 'name': '首相官邸(災害・危機管理情報)', 'screen_name': 'Kantei_Saigai', 'location': '', 'description': '本アカウントは首相官邸の公式アカウントです。\n首相官邸から災害・危機管理関連の政府活動情報をお届けいたします。', 'url': 'https://t.co/YlmtZlwbSB', 'entities': { 'url': { 'urls': [ { 'url': 'https://t.co/YlmtZlwbSB', 'expanded_url': 'https://www.kantei.go.jp/', 'display_url': 'kantei.go.jp', 'indices': [0, 23] } ] }, 'description': { 'urls': [] } }, 'protected': False, 'followers_count': 2836710, 'friends_count': 20, 'listed_count': 45036, 'created_at': 'Sun Mar 13 05:22:07 +0000 2011', 'favourites_count': 0, 'utc_offset': None, 'time_zone': None, 'geo_enabled': False, 'verified': True, 'statuses_count': 5741, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875550692454289408/Zfr37OhA_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875550692454289408/Zfr37OhA_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/265205959/1355386027', 'profile_link_color': '0084B4', 'profile_sidebar_border_color': 'C0DEED', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none' }, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': False, 'retweet_count': 5169, 'favorite_count': 5210, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja' }, 'is_quote_status': False, 'retweet_count': 5169, 'favorite_count': 0, 'favorited': False, 'retweeted': False, 'lang': 'ja' }, { 'created_at': 'Wed Apr 22 07:03:31 +0000 2020', 'id': 1252855534895521793, 'id_str': '1252855534895521793', 'text': 'RT @MofaJp_Sports: フィギュアスケート羽生結弦選手からのメッセージです????\nA video message from Hanyu Yuzuru, a Japanese figure skater. (Japanese only) \n#うちで過ごそう #St…', 'truncated': False, 'metadata': { 'iso_language_code': 'ja', 'result_type': 'recent' }, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': { 'id': 7080152, 'id_str': '7080152', 'name': 'Twitter Japan', 'screen_name': 'TwitterJP', 'location': '東京都中央区', 'description': '日本語版Twitter公式アカウントです。サービスに関してのご質問、お 問い合わせは https://t.co/mfQkUQLUhe および https://t.co/NZ3xoejW5Zをご利用ください。', 'url': 'https://t.co/veCH4MJbYL', 'entities': { 'url': { 'urls': [ { 'url': 'https://t.co/veCH4MJbYL', 'expanded_url': 'https://blog.twitter.com/ja_jp.html', 'display_url': 'blog.twitter.com/ja_jp.html', 'indices': [0, 23] } ] }, 'description': { 'urls': [ { 'url': 'https://t.co/mfQkUQLUhe', 'expanded_url': 'https://support.twitter.com/', 'display_url': 'support.twitter.com', 'indices': [42, 65] }, { 'url': 'https://t.co/NZ3xoejW5Z', 'expanded_url': 'https://support.twitter.com/articles/486421', 'display_url': 'support.twitter.com/articles/486421', 'indices': [70, 93] } ] } }, 'protected': False, 'followers_count': 2288941, 'friends_count': 140, 'listed_count': 15815, 'created_at': 'Tue Jun 26 01:54:35 +0000 2007', 'favourites_count': 988, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 13472, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': 'C0DEED', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': True, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875091517198614528/eeNe_9Pc_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/7080152/1493927138', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': 'FFFFFF', 'profile_sidebar_fill_color': 'DDEEF6', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': True, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'regular' }, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'retweeted_status': { 'created_at': 'Tue Apr 21 10:54:13 +0000 2020', 'id': 1252551207186386944, 'id_str': '1252551207186386944', 'text': 'フィギュアスケート羽生結弦選手からのメッセージです????\nA video message from Hanyu Yuzuru, a Japanese figure skater. (Japanese only)… https://t.co/dLkQH48VEo', 'truncated': True, ' { 'iso_language_code': 'ja', 'result_type': 'recent' }, 'source': '<a href="http://twitter.com/download/iphone" rel="nofollow">Twitter for iPhone</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': { 'id': 1153493906593501184, 'id_str': '1153493906593501184', 'name': '外務省MofaJapan×SPORTS', 'screen_name': 'MofaJp_Sports', 'location': 'Tokyo,Japan', 'description': 'SPORTS account of Ministry of Foreign Affairs of Japan. \n外務省のスポーツアカウント。スポーツ交流など外務省のスポーツを通じた様々な国際的取組みについて発信します。スポーツを通して外交を身近に!', 'url': 'https://t.co/E8nLgiaEbB', 'entities': { 'url': { 'urls': [ { 'url': 'https://t.co/E8nLgiaEbB', 'expanded_url': 'https://www.mofa.go.jp/mofaj/p_pd/ep/page24_000800.html', 'display_url': 'mofa.go.jp/mofaj/p_pd/ep/…', 'indices': [0, 23] } ] }, 'description': { 'urls': [] } }, 'protected': False, 'followers_count': 2106, 'friends_count': 284, 'listed_count': 57, 'created_at': 'Tue Jul 23 02:35:52 +0000 2019', 'favourites_count': 564, 'utc_offset': None, 'time_zone': None, 'geo_enabled': False, 'verified': True, 'statuses_count': 728, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': '000000', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/1217617971968741376/P0_I6cKy_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/1217617971968741376/P0_I6cKy_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/1153493906593501184/1578453418', 'profile_link_color': '1B95E0', 'profile_sidebar_border_color': '000000', 'profile_sidebar_fill_color': '000000', 'profile_text_color': '000000', 'profile_use_background_image': False, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none' }, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': True, 'quoted_status_id': 1251022418094649345, 'quoted_status_id_str': '1251022418094649345', 'quoted_status': { 'created_at': 'Fri Apr 17 05:39:22 +0000 2020', 'id': 1251022418094649345, 'id_str': '1251022418094649345', 'text': 'フィギュアスケートの羽生結弦選手からのメッセージです??\n#いまスポーツに できること #SportsAssistYou #Staystrong #Stayhome https://t.co/ASplkSQW7V', 'truncated': False, 'metadata': { 'iso_language_code': 'ja', 'result_type': 'recent' }, 'source': '<a href="https://mobile.twitter.com" rel="nofollow">Twitter Web App</a>', 'in_reply_to_status_id': None, 'in_reply_to_status_id_str': None, 'in_reply_to_user_id': None, 'in_reply_to_user_id_str': None, 'in_reply_to_screen_name': None, 'user': { 'id': 339200824, 'id_str': '339200824', 'name': '日本オリンピック委員会(JOC)', 'screen_name': 'Japan_Olympic', 'location': 'Tokyo, JAPAN', 'description': '日本オリンピック委員会(JOC)の公式ツイッターです。 Facebook: https://t.co/xgdGriW0ad Instagram: https://t.co/vb3VwM0hGF', 'url': 'https://t.co/LhygocfCSr', 'entities': { 'url': { 'urls': [ { 'url': 'https://t.co/LhygocfCSr', 'expanded_url': 'https://www.joc.or.jp/', 'display_url': 'joc.or.jp', 'indices': [0, 23] } ] }, 'description': { 'urls': [ { 'url': 'https://t.co/xgdGriW0ad', 'expanded_url': 'https://www.facebook.com/JapanOlympicTeam/', 'display_url': 'facebook.com/JapanOlympicTe…', 'indices': [38, 61] }, { 'url': 'https://t.co/vb3VwM0hGF', 'expanded_url': 'https://www.instagram.com/team_nippon/', 'display_url': 'instagram.com/team_nippon/', 'indices': [73, 96] } ] } }, 'protected': False, 'followers_count': 413875, 'friends_count': 534, 'listed_count': 2227, 'created_at': 'Wed Jul 20 18:52:37 +0000 2011', 'favourites_count': 1485, 'utc_offset': None, 'time_zone': None, 'geo_enabled': True, 'verified': True, 'statuses_count': 14321, 'lang': None, 'contributors_enabled': False, 'is_translator': False, 'is_translation_enabled': False, 'profile_background_color': '9AE4E8', 'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme16/bg.gif', 'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme16/bg.gif', 'profile_background_tile': False, 'profile_image_url': 'http://pbs.twimg.com/profile_images/875523921675665408/TDOMhMgV_normal.jpg', 'profile_image_url_https': 'https://pbs.twimg.com/profile_images/875523921675665408/TDOMhMgV_normal.jpg', 'profile_banner_url': 'https://pbs.twimg.com/profile_banners/339200824/1580371416', 'profile_link_color': '0084B4', 'profile_sidebar_border_color': 'BDDCAD', 'profile_sidebar_fill_color': 'DDFFCC', 'profile_text_color': '333333', 'profile_use_background_image': True, 'has_extended_profile': False, 'default_profile': False, 'default_profile_image': False, 'following': False, 'follow_request_sent': False, 'notifications': False, 'translator_type': 'none' }, 'geo': None, 'coordinates': None, 'place': None, 'contributors': None, 'is_quote_status': False, 'retweet_count': 14199, 'favorite_count': 30221, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja' }, 'retweet_count': 1069, 'favorite_count': 2607, 'favorited': False, 'retweeted': False, 'possibly_sensitive': False, 'lang': 'ja' }, 'is_quote_status': True, 'quoted_status_id': 1251022418094649345, 'quoted_status_id_str': '1251022418094649345', 'retweet_count': 1069, 'favorite_count': 0, 'favorited': False, 'retweeted': False, 'lang': 'ja' } ], 'search_metadata': { 'completed_in': 0.032, 'max_id': 0, 'max_id_str': '0', 'next_results': '?max_id=1252855534895521792&q=from%3ATwitterJP&geocode=&lang=&count=2&result_type=', 'query': 'from%3ATwitterJP', 'count': 2, 'since_id': 0, 'since_id_str': '0' } } |
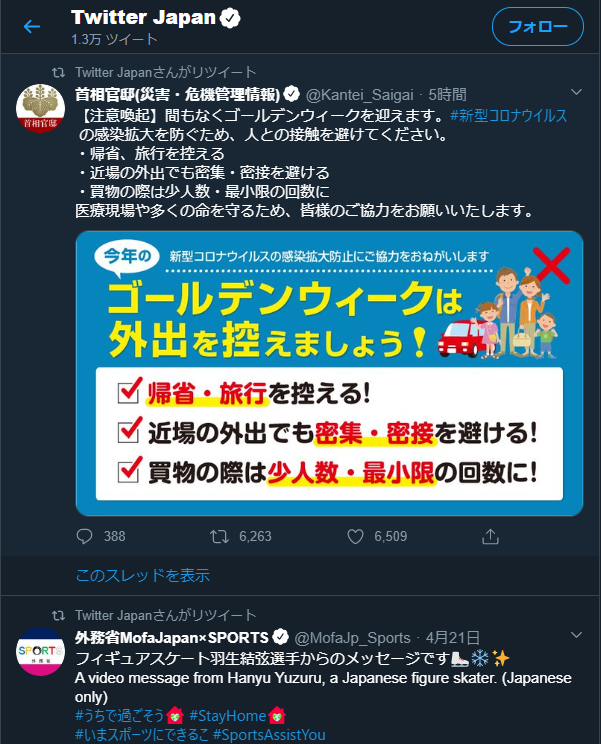
レスポンスから必要な情報を取得
dict(辞書)型とlist(リスト)型で構成されていることが分かりました
レスポンスから、欲しい情報だけ取得します
今回は、ツイート時刻(created_at)とツイート内容(text)を取得します
q項目を変えることで、気になるキーワードでツイートを検索したりすることが出来ます
今回は、プレーステーション公式アカウントの「FINAL FANTASY」のツイートを検索してみます
-filter:retweetsでリツートは検索対象外としています
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
# coding: utf_8 # ライブラリインポート import json from requests_oauthlib import OAuth1Session # APIキー&トークン設定 ConsumerAPIKey = 【ConsumerKey】 ConsumerAPIsecretkey = 【ConsumerSecretKey】 AccsessToken = 【AccessToken】 AceestokenSecret = 【AccessTokenSecret】 # 検索パラメータ設定 StandardSearchParameters = { 'q' : 'FINAL FANTASY from:PlayStation_jp -filter:retweets' ,'geocode' : '' ,'lang' : '' ,'locale' : '' ,'result_type' : '' ,'count' : '2' ,'until' : '' ,'since_id' : '' ,'max_id' : '' ,'include_entities' : '' } StandardSearchApiSession = OAuth1Session(ConsumerAPIKey, ConsumerAPIsecretkey, AccsessToken, AceestokenSecret) StandardSearchApiUrl = 'https://api.twitter.com/1.1/search/tweets.json' # 検索実行 StandardSearchResponse = StandardSearchApiSession.get(StandardSearchApiUrl, params = StandardSearchParameters) if StandardSearchResponse.status_code == 200: StandardSearchResult = json.loads(StandardSearchResponse.text) print ('1ツイート目') print ('【ツイート時刻】' + StandardSearchResult['statuses'][0]['created_at']) print ('【ツイート内容】' + StandardSearchResult['statuses'][0]['text']) print ("-----------\n2ツイート目") print ('【ツイート時刻】' + StandardSearchResult['statuses'][1]['created_at']) print ('【ツイート内容】' + StandardSearchResult['statuses'][1]['text']) |
1 2 3 4 5 6 7 8 9 10 11 |
$ python3 sample.py 1ツイート目 【ツイート時刻】Wed Apr 22 02:09:05 +0000 2020 【ツイート内容】『FINAL FANTASY VII REMAKE』の全世界累計販売本数が発売から3日で350万本を突破! 詳しくはこちら⇒ https://t.co/w99m7ZCgW0 #PS4 #FF7R https://t.co/OJKTk9PESC ----------- 2ツイート目 【ツイート時刻】Fri Apr 17 03:51:14 +0000 2020 【ツイート内容】【先週~今週発売の新作ゲーム】 「新たな物語」として生まれ変わった『FINAL FANTASY VII REMAKE』のほか、『Fallout 76: Wastelanders Deluxe Edition』や一風変わった横スクロ… https://t.co/Hj2kySKDuW |
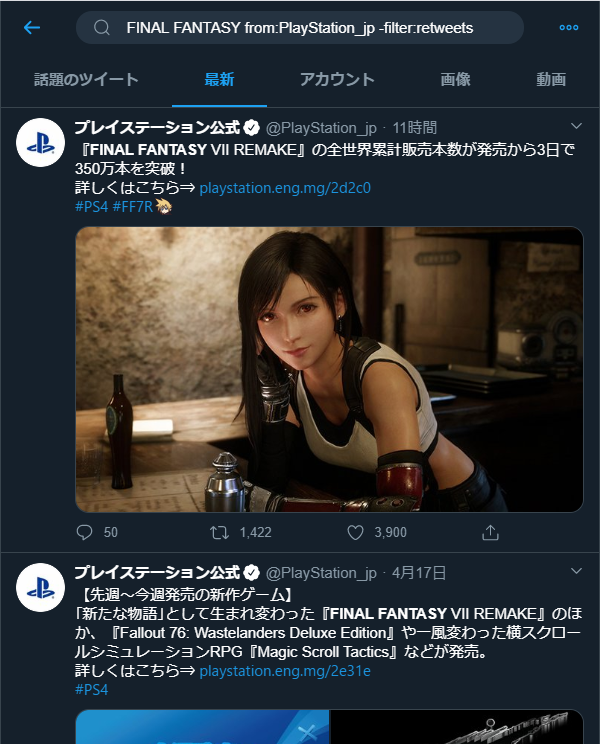
HTTPのレスポンスコードが200以外の場合
HTTPのレスポンスコードが200以外の場合も、textが返ってくる場合もあります
この場合、エラーに関する情報を取得出来ます
但し、エラー状況次第では、textがjson形式で読み込めない場合もあり、その場合はjson.loadでエクセプションが発生します
エクセプションに気を付ければ、必要に合わせてエラー原因の特定になる情報を確認出来ます
エラーコードについては、下記に情報があります
サンプルとして、認証エラーとなった場合の、エラー内容を確認してみます
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
# coding: utf_8 # ライブラリインポート import json from requests_oauthlib import OAuth1Session # APIキー&トークン設定 ConsumerAPIKey = 【誤ConsumerKey】 ConsumerAPIsecretkey = 【誤ConsumerSecretKey】 AccsessToken = 【誤AccessToken】 AceestokenSecret = 【誤AccessTokenSecret】 # 検索パラメータ設定 StandardSearchParameters = { 'q' : 'from:TwitterJP' ,'geocode' : '' ,'lang' : '' ,'locale' : '' ,'result_type' : '' ,'count' : '2' ,'until' : '' ,'since_id' : '' ,'max_id' : '' ,'include_entities' : '' } StandardSearchApiSession = OAuth1Session(ConsumerAPIKey, ConsumerAPIsecretkey, AccsessToken, AceestokenSecret) StandardSearchApiUrl = 'https://api.twitter.com/1.1/search/tweets.json' # 検索実行 StandardSearchResponse = StandardSearchApiSession.get(StandardSearchApiUrl, params = StandardSearchParameters) print ('【status_code】' + str(StandardSearchResponse.status_code)) print ('【reason】' + StandardSearchResponse.reason) StandardSearchResult = json.loads(StandardSearchResponse.text) print (StandardSearchResult) |
1 2 3 4 |
$ python3 sample.py 【status_code】401 【reason】Authorization Required {'errors': [{'code': 32, 'message': 'Could not authenticate you.'}]} |
[スポンサーリンク]
コメント